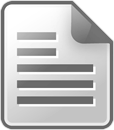
Here is a simple C example that demonstrates using stat(), posix_fadvise() and assert() for a file copy, not that its not great for sparse files, but this example is! ;)
/** * @file cpy.c * @author Ron Brash ([email protected] * @date Sept 12, 2014 * @brief Simple C program demonstrating posix fadvise for a file * copy application */ #include <stdio.h> #include <unistd.h> #include <sys/stat.h> #include <fcntl.h> #include <error.h> #include <string.h> #include <assert.h> #define BUF_SIZE 8192 // 8k ;) static off_t fsize(const char *filename); /** * fsize(const char *filename) * * @brief Returns the filesize of a file using stat.h * @param filename * @return -1 if there is an error, zero or positive value otherwise */ static off_t fsize(const char *filename) { struct stat st = { 0 }; if (stat(filename, &st) == 0) { return st.st_size; } return (-1); } int main(int argc, char **argv) { int inputFD = 0; int outputFD = 0; char buf[8192] = { }; ssize_t file_size = 0; /* Open a file descriptor for the input file */ if ((inputFD = open(argv[1], O_RDONLY)) == -1) { perror("Error opening input file descriptor"); return (-1); } /* Determine the file_size of the input file */ if ((file_size = fsize(argv[1])) < 0) { perror("Unable to determine accurate size of file"); return (-1); } /* Open a file descriptor for the output file */ if ((outputFD = open(argv[2], O_WRONLY | O_CREAT | O_TRUNC | O_APPEND, S_IRUSR | S_IWUSR)) == -1) { perror("Error opening output file descriptor"); return (-1); } /* Lets advise the kernel that we are going to attempt a long * sequential write */ if (posix_fadvise(inputFD, 0, 0, POSIX_FADV_SEQUENTIAL) < 0) { perror("Unable to advise the kernel for the upcoming sequential write"); // Continue anyways... } /* Read from the input file descriptor and write to the output file descriptor * while keeping the 8k within the CPUs L1 cache */ while (1) { file_size = read(inputFD, &buf[0], sizeof(buf)); if (!file_size) { break; } assert(file_size > 0); assert(write(outputFD, &buf[0], file_size) == file_size); } /* Close the input file descriptor */ if (close(inputFD) == -1) { perror("closing inputFD"); return (-1); } /* Close the output file descriptor */ if (close(outputFD) == -1) { perror("closing outputFD"); return (-1); } return 0; }
Blog tags:
Attachment | Size |
---|---|
![]() | 2.18 KB |
Add new comment