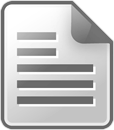
If you have been following the previous examples, you will note that I have only been copying a file, but not editing a file. One way to do this is to use lseek (or a sister function fseek). The below code will take an input file as the first argument, the second a file offset and the third some text to add.
-
/**
-
* @file seeker.c
-
* @brief File seeker that takes a file (argv[1]) and will write argv[3] at the
-
* position specified at argv[2]
-
*/
-
-
#define _FILE_OFFSET_BITS 64
-
#include <stdlib.h>
-
#include <stdio.h>
-
#include <fcntl.h>
-
#include <sys/stat.h>
-
#include <unistd.h>
-
#include <string.h>
-
-
int main (int argc, char **argv) {
-
-
int flags = O_RDWR | S_IRUSR | S_IWUSR;
-
int fd = 0;
-
off_t off = 0;
-
-
if((fd = open(argv[1], flags)) < 0 ) {
-
perror("Unable to open the input file");
-
return -1;
-
}
-
-
off = atoll(argv[2]);
-
-
if(lseek(fd, off, SEEK_SET) < 0) {
-
perror("Unable to open the input file");
-
return -1;
-
}
-
-
if (write(fd, argv[3], strlen(argv[3])) < 0 ) {
-
perror("unable to write to file \n");
-
return (-1);
-
}
-
-
close(fd);
-
-
return 0;
-
}
Now if you add the O_APPEND define to the flags argument like the bottom example - what happens?
-
/**
-
* @file append.c
-
* @brief File seeker that takes a file (argv[1]) and will write argv[3] at the
-
* position specified at argv[2]
-
*/
-
-
#define _FILE_OFFSET_BITS 64
-
#include <stdlib.h>
-
#include <stdio.h>
-
#include <fcntl.h>
-
#include <sys/stat.h>
-
#include <unistd.h>
-
#include <string.h>
-
-
int main (int argc, char **argv) {
-
-
int flags = O_RDWR | S_IRUSR | S_IWUSR | O_APPEND;
-
int fd = 0;
-
off_t off = 0;
-
-
if((fd = open(argv[1], flags)) < 0 ) {
-
perror("Unable to open the input file");
-
return -1;
-
}
-
-
off = atoll(argv[2]);
-
-
if(lseek(fd, off, SEEK_SET) < 0) {
-
perror("Unable to open the input file");
-
return -1;
-
}
-
-
if (write(fd, argv[3], strlen(argv[3])) < 0 ) {
-
perror("unable to write to file \n");
-
return (-1);
-
}
-
-
close(fd);
-
-
return 0;
-
}
The O_APPEND flag makes sure that the offset pointer within the file is ALWAYS at the end of the file regardless of where you placed the offset.
Add new comment