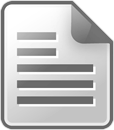
The other day I was playing around and required a quick and dirty C program which could be used for arrays or bucketing - it divides a value by a specified number and creates groups or teams.
Here it is in both a for loop and a while loop.
-
#include /<stdio.h/> //stdio and stdlib seem to get filtered
-
#include /<stdlib.h/>
-
-
/**
-
* Example program that takes a number, divides it by another specified
-
* number of your choice and then adds on the remainder (if it exists)
-
*
-
* To compile: gcc Whateveryoucallthis.c -o looper
-
*/
-
int
-
main(int argc, char **argv) {
-
-
int number = 39;
-
int divisor = 7;
-
int remainder = number % divisor;
-
int multi = number / divisor;
-
int fixed = number - remainder;
-
-
printf("Number: %d, fixed: %d, multi: %d, remainder %d\n",number,fixed,multi,remainder);
-
-
printf("For loop example \n");
-
-
// Initalize counters
-
int start = 0;
-
int end = 0;
-
int i = 0;
-
int x = 0;
-
-
for(i = 0; i< divisor; i++) {
-
-
x++;
-
start = end;
-
end = x*multi;
-
-
if( i == 6) {
-
end = end +remainder;
-
}
-
printf("%d.) start %d, end %d\n",i,start,end);
-
}
-
-
printf("While loop example \n");
-
-
// Reset counters
-
start = 0;
-
end = 0;
-
i = 0;
-
x = 0;
-
-
while(i < divisor) {
-
-
i++;
-
start = end;
-
end = i*multi;
-
-
if( i == divisor) {
-
end = end +remainder;
-
}
-
printf("%d.) start %d, end %d\n",i,start,end);
-
-
}
-
-
-
return 0;
-
}
Add new comment